C# Date/Time Property
using System
DateTime.Now;
Internationalization (I18n) Property Overview
The DateTime.Now property is the
DateTime object encapsulating
the current, local date and time on this computer.
See Microsoft's
MSDN online documentation for more information.
I18n Issues
Because DateTime
objects are culture-sensitive, developers retrieving
this property need to ensure that the
CultureInfo has been set
properly in the current thread, which would in turn ensure that the
DateTime.Now object was instantiated to use the correct
set of culture-specific rules.
It is also important to check code that uses the DateTime.Now
property to make sure that pieces of the current DateTime aren't being pasted
together in a locale-specific way, as in the case of the incorrect usage
example below.
You may also consider using DateTime.UtcNow rather than DateTime.Now ,
depending on how it's being used, since the time will be in
Universal Time (UTC) rather than in the system's local time zone.
Using DateTime.UtcNow.ToString("o") will result in a string that
is both time zone and locale independent,
e.g. 2008-06-15T21:15:07.0000000
Usage
The following example demonstrates the wrong way to use the
DateTime.Now property. This code example pieces together
a display date in a fashion that is specific to U.S. English. It will
be incorrect for even other English-speaking locales, such as the United
Kingdom.
string theMonth = DateTime.Now.Month.ToString();
string theDay = DateTime.Now.Day.ToString();
string theYear = DateTime.Now.Year.ToString();
string theTime = DateTime.Now.Hour.ToString() + ":" +
DateTime.Now.Minute.ToString() + ":" +
DateTime.Now.Second.ToString();
string displayTime = "Timestamp: " +
theTime + " " + theMonth + "/" + theDay + "/" + theYear;
The correct way to format a long date string such as the one
attempted above, would be to call the ToString method
on DateTime.Now , passing in the D format
string, which specifies a long date value. This way, if the correct
culture has been set in the current thread, the date formatting rules
of the user's culture will be used to generate the correct long-date
string. Even better, it's done in a single line of code instead of
eight:
string displayTime = DateTime.Now.ToString("D");
General C# date/time formatting information
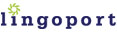
|